Sometimes it’s easier to pick the day’s orders all at once. This snippet adds a new menu item under WooCommerce called Pending Order Summary that prints a list of all the products needed to complete all pending orders.
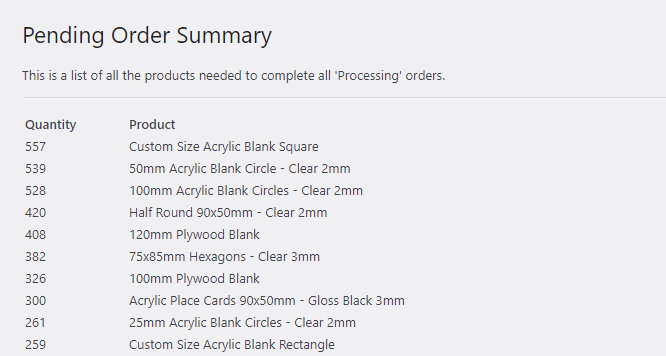
The plugin also has a helpful ‘Print’ button at the end of the list, which prints the list without any of the surrounding WordPress Dashboard.
function register_pending_order_summary_page() {
add_submenu_page( 'woocommerce', 'Pending Order Summary', 'Pending Order Summary', 'read', 'pending_order_summary_page', 'pending_order_summary_page_callback' );
}
function pending_order_summary_page_callback() { ?>
<div class="wrap">
<h1>Pending Order Summary</h1>
<p id="pendingordersubtitle">This is a list of all the products needed to complete all 'Processing' orders.<br /><hr /></p>
<?php
//Create an array to store order line items
$output_stack = array();
//Create an array to store order line item ids
$output_stack_ids = array();
//Array to display the results in numerical order
$output_diplay_array = array();
//SQL code
global $wpdb;
$order_line_items;
//Get the order ID of processing orders
$order_ids = $wpdb->get_results( $wpdb->prepare("SELECT ID FROM {$wpdb->prefix}posts where post_type = %s and post_status = %s;", 'shop_order', 'wc-processing' ));
//Collect the order IDs and add products to the pick list
foreach($order_ids as $an_order_id) {
//Collect all order line items matching IDs of pending orders
$order_line_items = $wpdb->get_results( $wpdb->prepare("SELECT * FROM {$wpdb->prefix}woocommerce_order_items where order_id = %d and order_item_type = %s;", $an_order_id->ID, 'line_item'));
//Extract the order line item
foreach($order_line_items as $line_item) {
//Add it to the array
$pair = array($line_item->order_item_name, $line_item->order_item_id);
array_push($output_stack, $line_item->order_item_name); //Now we've got a list of line items
array_push($output_stack_ids, $pair); //Now we've got a list of line ids
}
}
//Count the number of occurances of each value
$occurences = (array_count_values($output_stack));
// Print output
echo '<table><tr><td style="width: 100px;"><strong>Quantity</strong></td><td><strong>Product</strong></td></tr>';
if(count($occurences)>0){
$cumulative_count = 0;
//Loop through each different product in our list
foreach ($occurences as $product_name => $quantity) {
//Go through the list of ALL line items from earlier
foreach($output_stack_ids as $the_line_item) {
//We only want to cumulatively add the quantity if the line item name matches the current product name in the list of unique products
if ($product_name == $the_line_item[0]) {
//Reset the quanitity to zero, because we don't need to know the number of instances if we're counting stock
$quantity = 0;
//For each unique line item, we want to retrieve the quantity
$order_line_item_quantity = $wpdb->get_results( $wpdb->prepare("SELECT meta_value FROM {$wpdb->prefix}woocommerce_order_itemmeta where meta_key = %s and order_item_id=%d", "_qty", $the_line_item[1]));
//We've now got the quantity for each line item. Add them all together.
foreach($order_line_item_quantity as $item_quantity) {
//The total quantity is the combined _qty of each instance of a unique line item
$cumulative_count = $cumulative_count + ($item_quantity->meta_value );
}
}
}
$quantity = $cumulative_count;
$cumulative_count = 0;
$output_diplay_array[$product_name] = $quantity;
arsort($output_diplay_array);
}
//Output the results in a table
foreach ($output_diplay_array as $product_name => $quantity) {
echo '<tr><td>' . $quantity . '</td><td>' . $product_name.'</td></tr>';
}
echo '</table>';
//Some house cleaning
$wpdb->flush();
}
?>
<br /><a href="javascript:window.print()"><button type="button" class="orderprintbutton">Print</button>
</div>
<?php }
add_action('admin_menu', 'register_pending_order_summary_page',99);
//Function to apply print styling to the page
add_action('admin_head', 'dashboard_print_view');
function dashboard_print_view() {
echo '<style>
@media print {
#adminmenu{display: none!important;}
div#adminmenuback {display: none!important;}
#wpcontent {margin-left: 5%!important;}
#wpfooter {display: none!important;}
.orderprintbutton {display: none!important;"}
#screen-meta {display: none!important;}
}
</style>';
}
To install, add the above code to your theme’s functions.php file or to a MU Plugin. You can also use the Code Snippets plugin to install it, which is handy if you don’t have access to the filesystem.
Hi.
Your information has been very helpful.
Is it possible to add a line under each product or make it a table?
Thanks
Great help thankyou. Is it possible to just select from one category rather than the entire list?
Not easily. You’d need to grab the $product object associated with each line item and then use get_category_ids() to determine whether or not you want to include it in the list.
Awsome! Can we bring in extra columns? Like SKU and product Image, price?
Thanks a lot!!1
Would there be a way to make it go through and make this work for variations instead?
I’ve been looking but I’m honestly not 100% sure.
This does work for variable products. In the example screenshot, everything after the “-” is a variable product option.
Oh well I’m confused now. When I’m using this script it doesn’t seem to be separating the variations created using attributes to their own line items.
Seems like my replies to my messages aren’t working here.
So I worked out what’s wrong. It’s the products that have multiple variations that aren’t working properly.
Just wondering if there’s an easy way to fix this, unfortunately I’m not great when it comes to wordpress (I can do some modifications but don’t know that much)
Can we add a filter with the order date range?
Hi there 🙂 Thank you for the code, I have been using it for processing orders to do inventory. Is there a way to add SKU number to the table?
Thank you <3
– Libi
Thanks for this! Hope you post more Woocommerce tricks and hacks.
This is updated code with SKU and thumbnail:
https://pastebin.com/k3vdfgL9
Thanks for this! How can I list all of order IDs for one product?
Thanks for great article! How can I get the earlier date for an ID?