It’s very easy to produce a list of WooCommerce product tags, but limiting that list to just the tags in a specific product category is quite a bit more challenging.
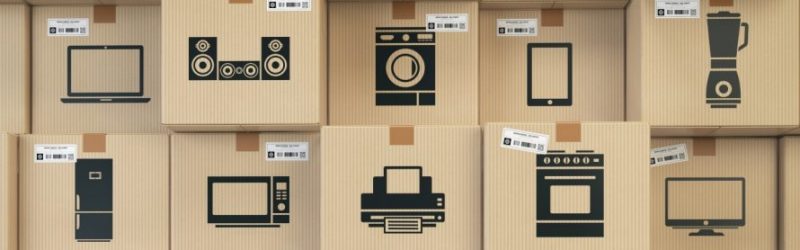
In order to achieve this, we need to do three things:
1. Loop through all of the products in the current category and add their tags to an array.
2. Filter the array to remove duplicates and then (optionally) sort it into alphabetical order.
3. Output the array into a list of links that filter the category by tag.
This code takes all of the tags from products in the current category and puts them into a combobox. It can be easily modified add the tags to a list by editing the HTML in the ‘//Output’ section.
//Get the current category (could also put the desired slug or id into $product_category directly)
$term = get_queried_object();
$product_category = $term->slug;
//Iterate through all products in this category
$query_args = array(
'product_cat' => $product_category,
'post_type' => 'product',
//Grabs ALL post
'posts_per_page' => -1
);
$query = new WP_Query( $query_args );
$term_array = array();
while( $query->have_posts() ) {
$query->the_post();
$terms = get_the_terms( get_the_ID(), 'product_tag' );
if ( ! empty( $terms ) && ! is_wp_error( $terms ) ){
foreach ( $terms as $term ) {
$term_array[] = $term->name;
}
}
}
//Remove any duplicates.
$tags_unique = array_unique($term_array);
//Sort alphabetically
asort($tags_unique);
//Output
echo '<select onchange="window.location=this.options[this.selectedIndex].value">';
echo '<option value="Filter by Tag">Filter by Tag</option>';
foreach($tags_unique as $unique) {
//it's faster to "guess" the tag slug by replacing spaces with dashes and stripping special chars
$special_characters = array("=", "+", "/", "'",")","(");
$tag_slug = str_replace(" ","-",$unique);
$tag_slug = strtolower(str_replace($special_characters,"",$tag_slug));
echo '<option value="https://www.yourdomain.com/category/' . $product_category .'/?product_tag='. $tag_slug .'"> '. $unique . '</option>';
}
echo '</select>';
//Reset the query
wp_reset_postdata();
WooCommerce does all of the heavy lifting for the actual product filtering. Appending ‘?product_tag=your_tag’ to the end of the URL is enough to only show products with a certain tag within a specified category.
One of our clients–a pet supply wholesaler–uses product tags to store the manufacturer/brand of each product. This filter makes it simple for their customers to filter product categories by brand.
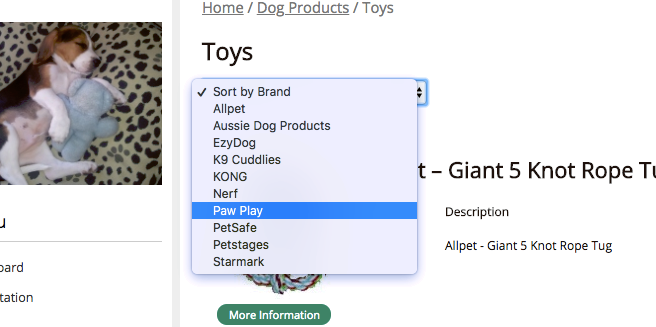
It should be noted that iterating through every product in a category to retrieve the tags is a resource-intensive task, especially on stores with a lot of products in each category. Page caching or better hosting might be necessary if you find this slows your site down too much.
If you find any issues with this code, or have any ideas to improve its performance, please let me know in the comments.
Thank very much man, you saved me.
it is smart and mobile friendly. thanks a lot. I am very happy 😀